Honeycomb.io (Tracing)
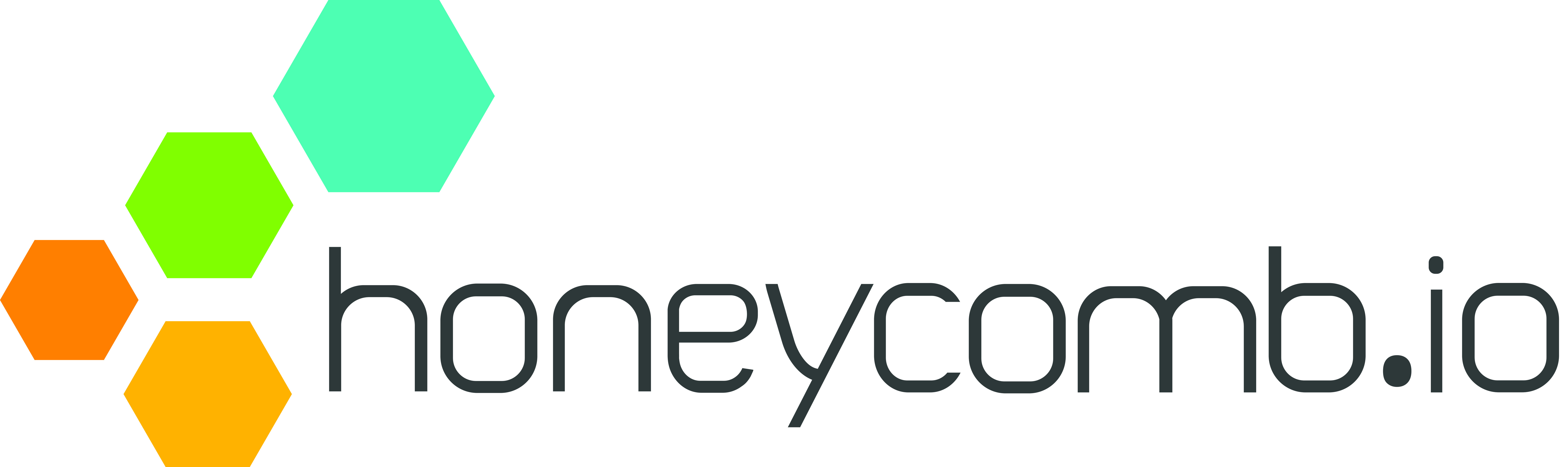
Introduction
Honeycomb is a service for debugging your software in production. Capture traces or individual events, then speedily slice and dice your data to uncover patterns, find outliers, and understand historical trends.
Its OpenCensus Python exporter is available at https://github.com/codeboten/ochoneycomb
Installing the exporter
The Honeycomb Python exporter can be installed from pip by
pip install ochoneycomb
Creating the exporter
To create the exporter, we’ll need to:
- Create an exporter in code
- Pass in your Honeycomb API key, found on your Honeycomb Team Settings page. (Sign up for free if you haven’t already!)
- Pass in your Honeycomb dataset name
import os
import time
from ochoneycomb import HoneycombExporter
from opencensus.trace.tracer import Tracer
exporter = HoneycombExporter(
writekey=os.getenv("HONEYCOMB_WRITEKEY"),
dataset=os.getenv("HONEYCOMB_DATASET"),
service_name="test-app")
tracer = Tracer(exporter=exporter)
def do_something_to_trace():
time.sleep(1)
# Example for creating nested spans
with tracer.span(name="span1") as span1:
do_something_to_trace()
with tracer.span(name="span1_child1") as span1_child1:
span1_child1.add_annotation("something")
do_something_to_trace()
with tracer.span(name="span1_child2") as span1_child2:
do_something_to_trace()
with tracer.span(name="span2") as span2:
do_something_to_trace()
Viewing your traces
Please visit the Honeycomb UI to view your traces.
Learn more about exploring your trace data here.
References
Resource | URL |
---|---|
Honeycomb exporter on pip | https://pypi.org/project/ochoneycomb/ |
Honeycomb exporter on Github | https://github.com/codeboten/ochoneycomb |